Blogs
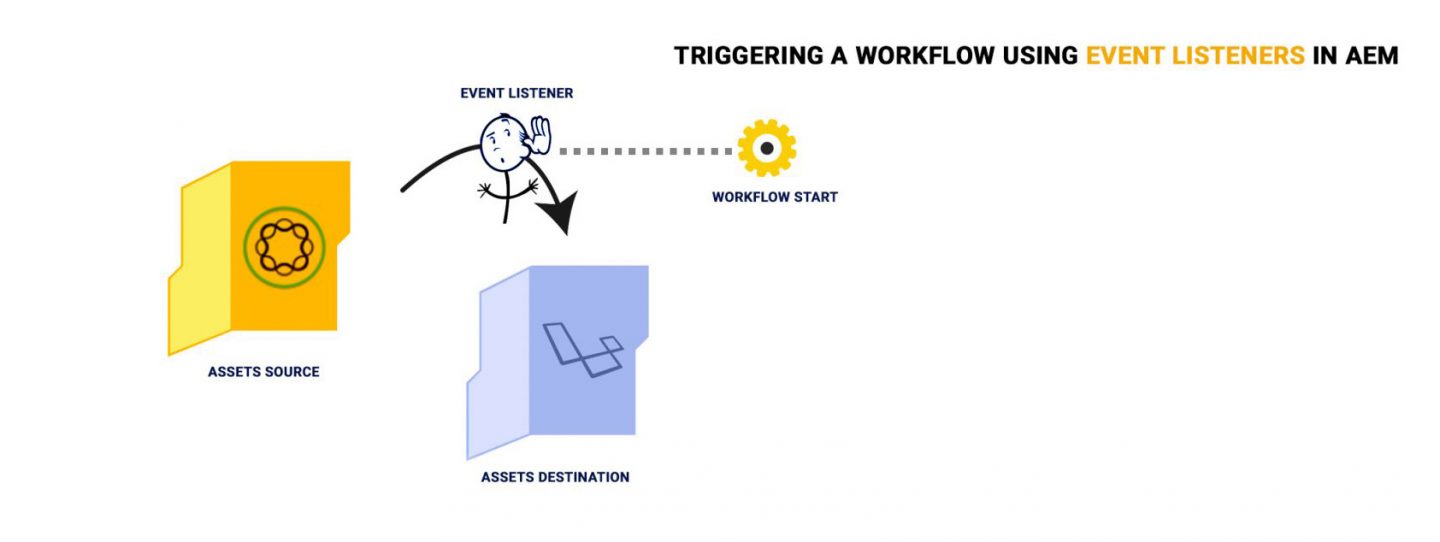
Trigger a Workflow in AEM
We can trigger a workflow in AEM 6.2 when a DAM Asset is created, modified, or deleted within a given path. In this article, we will explore triggering workflows from our code based on events in the JCR.
Suppose you have a workflow that creates custom renditions of assets in addition to the default AEM renditions, when the asset is under “/content/dam/ProjectName/images/”. You would have set up two workflow launchers for triggering this workflow: one with event type as “Node Create” and one with “Node Modified”. We can also achieve the same functionality through our code, without touching the GUI.
Requirements
When assets are moved into a certain folder structure in DAM, trigger a workflow that creates a 100px X 100px thumbnail of our image.
Fig 1: Before Moving the asset, no custom thumbnail. Fig 2: Desired result after moving the asset, the new thumbnail.
Analysis
The intuitive thought is that when an asset is moved, a new node is created in the new location and the old one is deleted. However, experience shows that AEM does not create a new node in the destination folder on Node Move. We know this because the ‘jcr:Created’ property does not change. AEM does not even change the last modified date.
Fig 3: Creation Timestamp Before Moving the Asset. Fig 4: Creation Timestamp is the same after moving.
Fig 5: Modification Timestamp Before Moving the Asset. Fig 6: Modification Timestamp is the same after moving.
What if we copy the asset?
On copying the asset, a new version of the same is created. This triggers the Node Creation launcher.
Fig. 7: No versions before copying the asset. Fig. 8: Version created after copy-pasting the asset.
Approach
Event Listeners
AEM supports observation, which enables us to receive notifications of persistent changes to the workspace. A persisted change to the workspace is represented by a set of one or more events. Each event reports a single simple change to the structure of the persistent workspace in terms of an item added, changed, moved or removed. There are thus 7 possible events at the JCR level, viz:
- Node Added
- Node Moved
- Node Modified
- Node Removed
- Property Added
- Property Removed
- Property Changed
We connect with the observation mechanism by registering an event listener with the workspace. An event listener is a class implementing the EventListener interface, that responds to the stream of events to which it has been subscribed. An event listener is added to a workspace with:
void ObservationManager. addEventListener(EventListener listener, int eventTypes, String absPath, boolean isDeep, String[] uuid, String[] nodeTypeName, boolean noLocal)
(A detailed explanation of each parameter is given with the code example in the package as well as at the end of this article) As defined by the EventListener interface, listener must provide an implementation of the onEvent method:
void EventListener.onEvent(EventIterator events)
When an event occurs that falls within the scope of the listener, the repository calls the onEvent method invoking our logic which processes/responds to the event. In our case, we will register an event listener to listen for “Node Moved” events under “/content/dam/images” so that when an asset is moved to that folder, our workflow can be triggered.
Implementation
When the component is activated, the activate(…) method is called. It contains a call to ObservationManager.addEventListener(…) for registering the event listener. The deactivate(…) method contains logic for deregistering the event listener, and is triggered when the bundle is being stopped.
When the relevant event occurs, the onEvent(…) method is called, which contains logic for processing the event. In our case, we trigger a workflow in AEM.
The following is the relevant code from ThumbnailNodeMovedListener.java:
protected void activate(ComponentContext ctx) { try { . . . // Building the parameters for adding the event listener // Whether the subfolders of the given path should also be watched boolean isDeep = true; // Only events whose associated node has one of the UUIDs in this list will be // received. If this parameter is null then no UUID-related restriction is // placed on events received. String[] uuid = null; // Only events whose associated node has one of the node types (or a subtype of // one of the node types) in this list will be received. If this parameter is // null then no node type-related restriction is placed on events received. String[] nodeTypeName = null; // If noLocal is true, then events generated by the session through which the // listener was registered are ignored. Otherwise, they are not ignored. boolean noLocal = true; // Registering the event listener observationManager.addEventListener(this, Event.NODE_MOVED, ASSET_UPDATE_PATH, isDeep, uuid, nodeTypeName, noLocal); } } public void onEvent(EventIterator itr) { while (itr.hasNext()) { Event currentEvent = itr.nextEvent(); try { . . . // Create a workflow session WorkflowSession wfSession = workflowService.getWorkflowSession(localSession); // Get the workflow model WorkflowModel wfModel = wfSession.getModel(THUMBNAIL_WORKFLOW_PATH); // Get the Workflow data. The first parameter in the newWorkflowData method is // the payloadType. Just a fancy name to let it know what type of workflow it is // working with. WorkflowData wfData = wfSession.newWorkflowData(JCR_PATH, currentEvent.getPath()+ORIGINAL_RENDITION_RELATIVE_PATH); // Start the Workflow. wfSession.startWorkflow(wfModel, wfData); } . . .
Download this code (including the workflow):
Build it using
mvn clean install -PautoInstallPackage
N.B: Creating a workflow is not part of this tutorial, and therefore a ready workflow has been provided in the code package. However, if you want to learn how to create custom workflows, here is an excellent resource.
References
Adobe Consulting Services. (2018, March 20). acs-aem-samples/SampleJcrEventListener.java at master · Adobe-Consulting-Services/acs-aem-samples. Retrieved from Github: https://github.com/Adobe-Consulting-Services/acs-aem-samples/blob/master/bundle/src/main/java/com/adobe/acs/samples/events/impl/SampleJcrEventListener.java
Day Software AG. (2018, March 20). JCR 2.0: 12 Observation (Content Repository for Java Technology API v2.0). Retrieved from Adobe Docs: https://docs.adobe.com/docs/en/spec/jcr/2.0/12_Observation.html
FAQs
- What is an event listener?
An event listener is a procedure or function in a program that waits for an event to occur. - What are some examples of an event in a computer program?
Some simple examples of an event are users clicking or moving the mouse, pressing a key on the keyboard, or network activity. - What are workflow launchers?
A workflow launcher is a standard way to invoke or trigger a workflow based on conditions. The workflow launcher monitors changes in the content repository to launch workflows dependent on the location and resource type of the changed node.